There is a tray of items in camera space at the top of the game window at
all times. The items in this tray rotate around the y-axis to create an
arcade game like aesthetic.
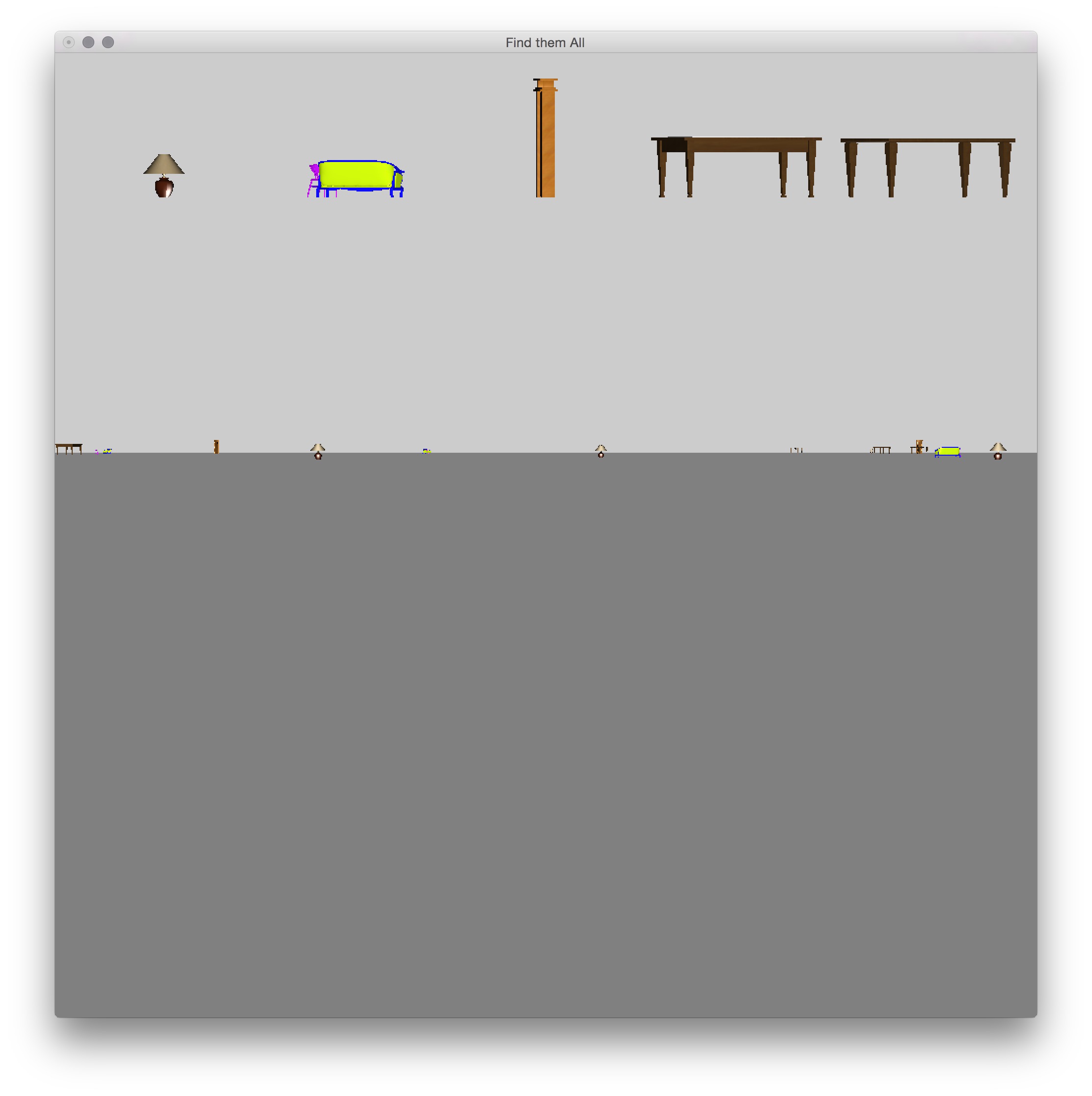
To find an object in the world, simple bounding box collision detection has
been implemented. If the camera's world coordinates are ever inside the on
the edges of or inside an objects bounding box, then that object will be "found".
To indicate that this has happened, the object will explode into 100 smaller,
randomly oriented copies of itself and move upward and outward.
This explosion will last for 240 frames, and then disappear. Once the
explosion has finished, the item tray will be notified that the object has
been found. If it so happens that the object found is the last instance
of it in the world, then it will be removed from the item tray.
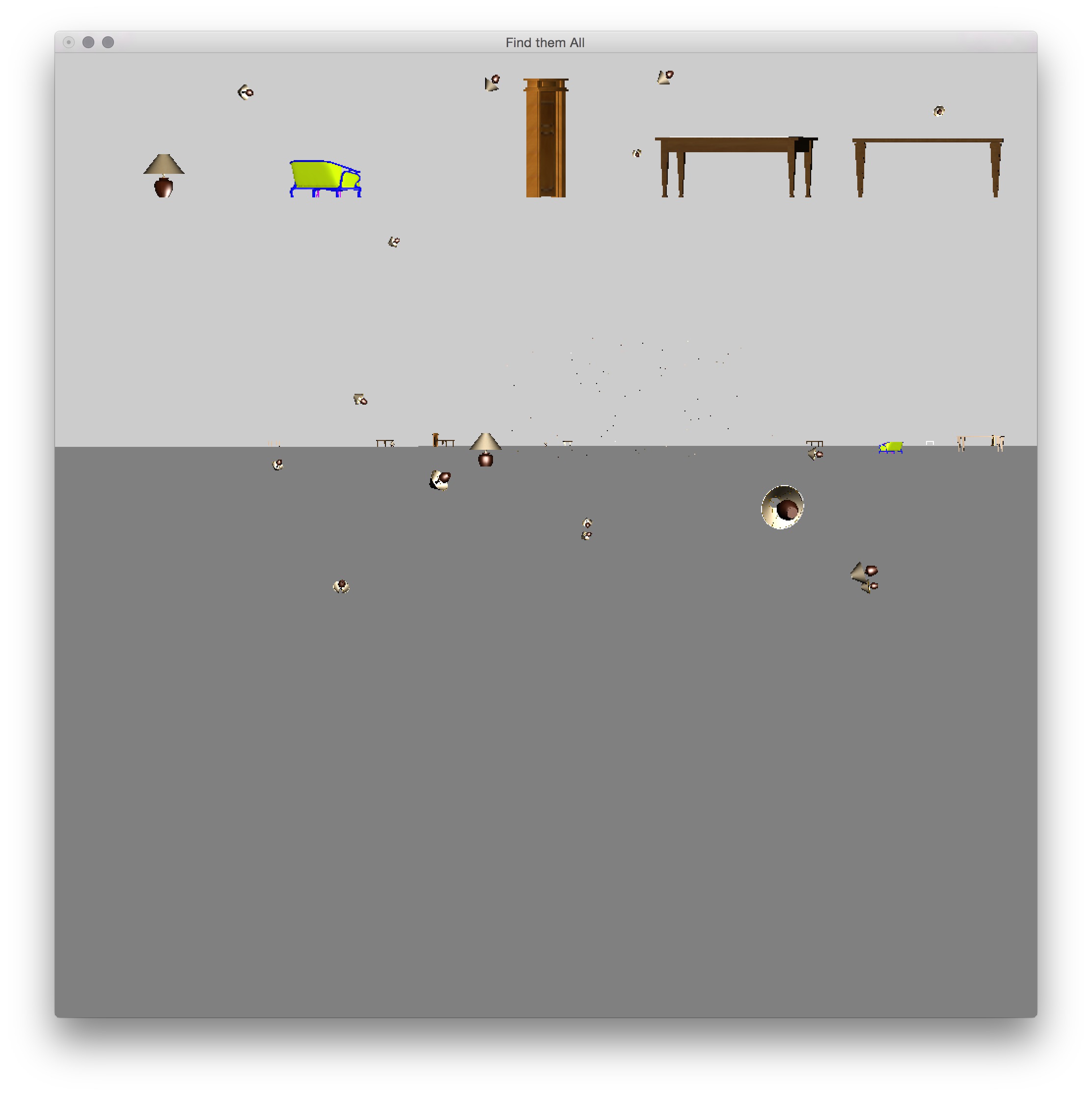
Once all the instances of the items in the item tray have been found, the
world will be re-randomized and a new set of objects to find will be selected.
The game is never-ending.